In Coding Page
<asp:GridView ID="GridView1" runat="server" Style="z-index: 100; left: 102px; position: absolute;top: 51px" AutoGenerateColumns="False" AutoGenerateDeleteButton="True" AutoGenerateEditButton="True" DataKeyNames="eno" OnRowCancelingEdit="GridView1_RowCancelingEdit" OnRowCreated="GridView1_RowCreated" OnRowDeleting="GridView1_RowDeleting" OnRowEditing="GridView1_RowEditing" OnRowUpdating="GridView1_RowUpdating" ShowFooter="True" CellPadding="4" ForeColor="#333333" GridLines="None">
<Columns>
<asp:BoundField DataField="eno" HeaderText="EmpNo" ReadOnly="True" SortExpression="eno" />
<asp:BoundField DataField="ename" HeaderText="ename" SortExpression="ename" />
<asp:BoundField DataField="sal" HeaderText="sal" SortExpression="sal" />
Columns>
<FooterStyle BackColor="#507CD1" Font-Bold="True" ForeColor="White" />
<RowStyle BackColor="#EFF3FB" />
<EditRowStyle BackColor="#2461BF" />
<SelectedRowStyle BackColor="#D1DDF1" Font-Bold="True" ForeColor="#333333" />
<PagerStyle BackColor="#2461BF" ForeColor="White" HorizontalAlign="Center" />
<HeaderStyle BackColor="#507CD1" Font-Bold="True" ForeColor="White" />
<AlternatingRowStyle BackColor="White" />
asp:GridView>
<asp:Button ID="btninsert" runat="server" OnClick="btninsert_Click" Text="Add" Width="68px" style="z-index: 102; left: 103px; position: absolute; top: 25px" />
Set The Following Values in GridView Properties
AutoGenerateColumes=false;
AutoGenerateDelete=True;
AutoGenerateEditButton=true;
Datakeynames=eno;(Primary key coloume name)
ShowFooter=True;
The Following example is used MySql DB;
Example table Is Emp1(eno,ename,sal fields)
In .cs File
using System;
using System.Data;
using System.Configuration;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.Data.Odbc;
public partial class _Default : System.Web.UI.Page
{
string str;
OdbcConnection myConn
= new OdbcConnection("driver={Mysql odbc 3.51 driver};user id=root;password=admin;server=localhost;database=temp");
protected void Page_Load(object sender, EventArgs e)
{
if (IsPostBack == false)
{
str = "select * from emp1";
getdata(str);
}
}
public void getdata(string s)
{
OdbcDataAdapter da = new OdbcDataAdapter(s, myConn);
DataSet ds = new DataSet();
da.Fill(ds, "raji");
GridView1.DataSource = ds;
GridView1.DataBind();
}
protected void GridView1_RowDeleting(object sender, GridViewDeleteEventArgs e)
{
int row = Convert.ToInt32(GridView1.DataKeys[e.RowIndex].Value);
OdbcCommand cmd = new OdbcCommand("delete from emp1 where eno=" + row, myConn);
myConn.Open();
cmd.ExecuteNonQuery();
myConn.Close();
getdata("select * from emp1");
}
protected void GridView1_RowUpdating(object sender, GridViewUpdateEventArgs e)
{
GridViewRow row;
row = GridView1.Rows[e.RowIndex];
TextBox t;
t = (TextBox)row.Cells[2].Controls[0];
string nename = t.Text;
t = (TextBox)row.Cells[3].Controls[0];
int nsal = int.Parse(t.Text);
int eno = Convert.ToInt32(GridView1.DataKeys[e.RowIndex].Value);
OdbcCommand cmd = new OdbcCommand("update emp1 set ename='" + nename + "',sal=" + nsal + " where eno=" + eno, myConn);
myConn.Open();
cmd.ExecuteNonQuery();
myConn.Close();
GridView1.EditIndex = -1;
getdata("select * from emp1");
}
protected void GridView1_RowEditing(object sender, GridViewEditEventArgs e)
{
GridView1.EditIndex = e.NewEditIndex;
getdata("select * from emp1");
}
protected void GridView1_RowCancelingEdit(object sender, GridViewCancelEditEventArgs e)
{
GridView1.EditIndex = -1;
getdata("select * from emp1");
}
protected void GridView1_RowCreated(object sender, GridViewRowEventArgs e)
{
if (e.Row.RowType == DataControlRowType.Footer)
{
TableCell cell1 = e.Row.Cells[1];
TextBox txteno = new TextBox();
txteno.ID = "txteno";
txteno.Width = 70;
cell1.Controls.Add(txteno);
TableCell cell2 = e.Row.Cells[2];
TextBox txtename = new TextBox();
txtename.ID = "txtename";
txtename.Width = 70;
cell2.Controls.Add(txtename);
TableCell cell3 = e.Row.Cells[3];
TextBox txtsal = new TextBox();
txtsal.ID = "txtsal";
txtsal.Width = 70;
cell3.Controls.Add(txtsal);
}
}
protected void btndelete_Click(object sender, EventArgs e)
{
string ds = "";
foreach (GridViewRow r in GridView1.Rows)
{
CheckBox cb = (CheckBox)r.FindControl("chk1");
if (cb.Checked)
{
int eno = Convert.ToInt32(GridView1.DataKeys[r.RowIndex].Value);
ds = "delete from emp1 where eno=" + eno;
OdbcCommand cmd = new OdbcCommand(ds, myConn);
myConn.Open();
cmd.ExecuteNonQuery();
myConn.Close();
}
}
getdata("select * from emp1");
}
protected void btninsert_Click(object sender, EventArgs e)
{
GridViewRow r = GridView1.FooterRow;
TextBox t;
t = (TextBox)r.FindControl("txteno");
int eno = int.Parse(t.Text);
t = (TextBox)r.FindControl("txtename");
string ename = t.Text;
t = (TextBox)r.FindControl("txtsal");
int sal = int.Parse(t.Text);
OdbcCommand cmd = new OdbcCommand("insert into emp1 values(" + eno + ",'" + ename + "'," + sal + ")", myConn);
myConn.Open();
cmd.ExecuteNonQuery();
cmd.Dispose();
getdata("select * from emp1");
}
}
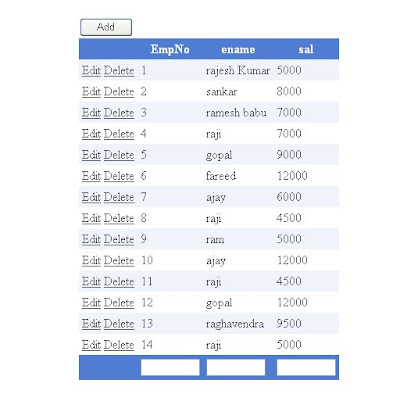
5 comments:
hi.i use this code. but when i click on edit and then change the values, and then click on the update button then it do not update the value.Instead it shows the old value.
CType((row.Cells[2].Controls[0],TextBox).Textthis gives me the old value not updated.
Top web site, I had not noticed funnydotnet.blogspot.com earlier in my searches!
Keep up the fantastic work!
Thanks for sharing the link, but unfortunately it seems to be down... Does anybody have a mirror or another source? Please answer to my post if you do!
I would appreciate if a staff member here at funnydotnet.blogspot.com could post it.
Thanks,
Mark
Greetings,
This is a question for the webmaster/admin here at funnydotnet.blogspot.com.
Can I use some of the information from your post right above if I give a link back to your website?
Thanks,
James
James..
You can use require info with a Back Link.
Cheers...
Respects for your's Questions & Opinions